5 things you didn't know about WebAssembly
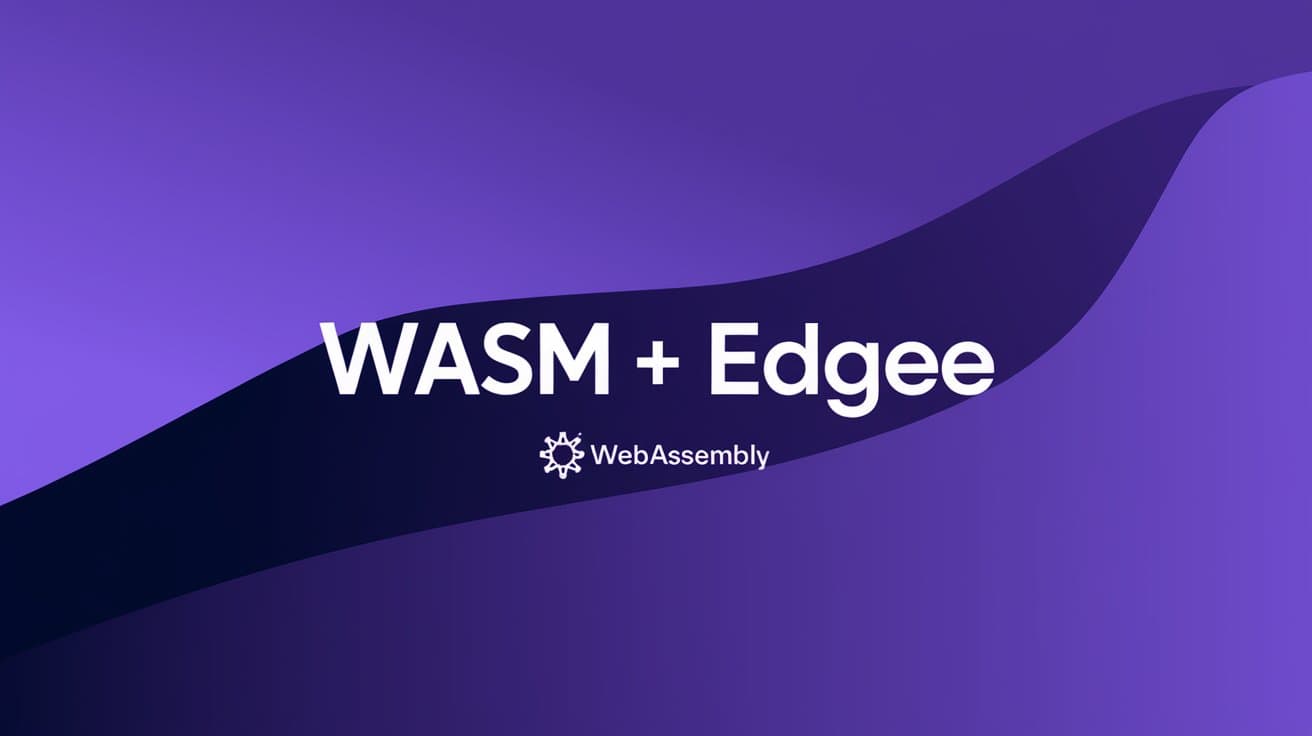
WebAssembly (Wasm) is emerging as a transformative technology, enabling developers to run code efficiently across platforms. It offers remarkable portability, performance, and security features, making it a compelling choice for modern application development.
In this article, we'll explore the technical aspects of WebAssembly and share some useful resources and insights.
1. Cross-platform portability of Wasm binaries
One of WebAssembly's standout features is its ability to run the same binary code across different operating systems without modifications. This cross-platform portability simplifies deployment and ensures consistent behavior across environments.
Under the hood, WebAssembly achieves portability through a well-defined binary format and execution semantics: you compile source code from languages like C, C++, or Rust into a .wasm binary. Then, you can run this binary on any platform with a compatible Wasm runtime, such as Wasmtime.
Example: compiling a C++ function to WebAssembly
Consider a simple C++ function that prints to stdout:
// hello.cpp
#include <stdio.h>
int main() {
printf("hello world from Edgee!\n");
return 0;
}
You can compile this function to WebAssembly with a tool such as Emscripten:
$ emcc hello.cpp -O3 -o hello.wasm
And then run it with any Wasm runtime, on any operating system.
For example, with wasmtime:
$ wasmtime hello.wasm
hello world from Edgee!
This approach also works across different programming languages, thanks to native support and tooling. We will explore that later in this article.
Wasm binary manipulation
You can also use utilities such as wasm-tools to validate, manipulate, optimize, and compose binary files.
Let's reuse the hello.wasm file we generated earlier and have a look at some sample commands.
We could generate its textual representation to stdout:
$ wasm-tools print hello.wasm
Or remove custom sections to minimize its size (in this case there isn't much to strip):
$ wasm-tools strip hello.wasm -o hello-stripped.wasm
Add custom metadata (OCI-compliant):
$ wasm-tools metadata add hello.wasm \
--name "Hello world test" \
--authors "Alex Casalboni" \
--source https://www.edgee.cloud/blog/web-assembly \
--version 1.0.0 \
--sdk emscripten=4.0.3 \
-o hello-with-metadata.wasm
And log metadata to stdout (useful with more complex binaries, especially when working with components):
$ wasm-tools metadata show hello-with-metadata.wasm
╭─────────┬───────────────────────────────────────────╮
│ KIND ┆ VALUE │
╞═════════╪═══════════════════════════════════════════╡
│ kind ┆ module │
├╌╌╌╌╌╌╌╌╌┼╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌┤
│ name ┆ Hello world test │
├╌╌╌╌╌╌╌╌╌┼╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌┤
│ range ┆ 0x0..0x89a │
├╌╌╌╌╌╌╌╌╌┼╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌┤
│ authors ┆ Alex Casalboni │
├╌╌╌╌╌╌╌╌╌┼╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌┤
│ source ┆ https://www.edgee.cloud/blog/web-assembly │
├╌╌╌╌╌╌╌╌╌┼╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌┤
│ version ┆ 1.0.0 │
├╌╌╌╌╌╌╌╌╌┼╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌╌┤
│ sdk ┆ emscripten [4.0.3] │
╰─────────┴───────────────────────────────────────────╯
2. Near-native performance
WebAssembly is designed for high performance, often achieving execution speeds close to native code, or even faster. This comes from its compact binary format and the ability to be JIT-compiled by host environments.
Benchmarks indicate that WebAssembly code runs at 80-90% of native speed in many scenarios. This is particularly beneficial for compute-intensive applications such as gaming, image processing, and scientific simulations.
In addition to enabling applications that would typically struggle to run in the browser, Wasm is also highly relevant for server-side use cases, as we’ll explore later.
Example: Performance Comparison with Node.js
We ran some benchmarks based on this repository that compares WebAssembly to native Node.js implementations (and C++ Addons) of various algorithms such as Levenshtein distance, Fibonacci, Fermat primality test, linear regression, and SHA256.
Levenshtein-Distance:
Native x 702,634 ops/sec ±0.20% (101 runs sampled)
N-API Addon x 999,204 ops/sec ±0.06% (101 runs sampled)
WebAssembly x 782,177 ops/sec ±2.41% (99 runs sampled)
Fastest: N-API Addon
# TL;DR: Wasm is 11% faster than native and 22% slower than C++ Addon
Fibonacci:
Native x 10,647,107 ops/sec ±0.09% (100 runs sampled)
N-API Addon x 25,974,647 ops/sec ±0.82% (93 runs sampled)
WebAssembly x 39,514,938 ops/sec ±0.52% (96 runs sampled)
Fastest: WebAssembly
# TL;DR: Wasm is 271% faster than native and 52% faster than C++ Addon
Fermat-Primality-Test:
Native x 1,024,513 ops/sec ±0.10% (101 runs sampled)
N-API Addon x 9,986,498 ops/sec ±0.40% (98 runs sampled)
WebAssembly x 14,743,231 ops/sec ±0.11% (98 runs sampled)
Fastest: WebAssembly
# TL;DR: Wasm is 1339% faster than native and 47% faster than C++ Addon
Simple-Linear-Regression:
Native x 638,257 ops/sec ±0.43% (95 runs sampled)
N-API Addon x 15,221 ops/sec ±0.76% (98 runs sampled)
N-API Addon using TypedArrays x 252,743 ops/sec ±0.05% (100 runs sampled)
WebAssembly x 149,045 ops/sec ±0.07% (101 runs sampled)
WebAssembly using TypedArrays x 159,803 ops/sec ±0.32% (102 runs sampled)
Fastest: Native
# TL;DR: Wasm is 299% slower than native and 37% slower than C++ Addon
SHA256:
Native x 46,664 ops/sec ±0.28% (98 runs sampled)
N-API Addon x 325,056 ops/sec ±0.28% (100 runs sampled)
WebAssembly x 152,833 ops/sec ±0.75% (97 runs sampled)
Fastest: N-API Addon
# TL;DR: Wasm is 227% faster than native and 53% slower than C++ Addon
To summarize: excluding linear regression, Wasm is always faster than native. For some use cases, between 3x and 15x faster than native.
Similar considerations apply to other interpreted languages such as Python, where compiling to Wasm often requires bundling the entire interpreter.
3. Lightweight and Fast Initialization
Wasm modules are known for their small size and rapid startup times, making them ideal for resource-constrained environments or scenarios requiring quick initialization.
A typical Wasm module ranges from 100 to 500 KB, significantly smaller than traditional container images, which can easily reach several megabytes or even gigabytes in size. Additionally, Wasm modules can initialize in milliseconds, enabling responsive applications and services.
Example: Deploying an HTTP Wasm module
Using a platform like Fermyon Spin, you can build and deploy a Wasm module with minimal overhead:
spin new myapp -t http-rust
cd myapp
spin build
ls -lart target/wasm32-wasip1/release/myapp.wasm
-rwxr-xr-x 1 alex staff 308140 Feb 20 13:14 target/wasm32-wasip1/release/myapp.wasm
# TL;DR: the Wasm file is only 300KB!
4. Secure Sandboxing
Security is the top priority for code execution, especially when running untrusted code. Wasm addresses this by executing code within a memory-safe sandbox, restricting unauthorized access to network and file system.
In other words, the WebAssembly runtime enforces strict boundaries, preventing code from performing arbitrary system calls or accessing the file system unless explicitly permitted.
This containment makes it an excellent choice for executing untrusted code safely.
Example: Running untrusted code
Consider a scenario where you need to run 3rd-party code securely. By executing the code within a Wasm runtime like Wasmtime, you can ensure it operates within an isolated environment.
Here is what the Rust code would look like:
use wasmtime::*;
fn main() -> Result<()> {
// Create an engine and a store
let engine = Engine::default();
let store = Store::new(&engine, MyApplicationState{...});
// Load Wasm module from file
let module = Module::from_file(&engine, "untrusted.wasm")?;
// Instantiate the module
let instance = Instance::new(&store, &module, &[])?;
// Invoke a `run` function from the module
let run = instance.get_typed_func::<(), ()>(&mut store, "run")?;
run.call(&mut store, ())?;
Ok(())
}
In this example, the untrusted.wasm module runs within a controlled environment, mitigating potential security risks.
5. Multi-language support and versatility
The WebAssembly design supports a wide range of programming languages, enabling developers to leverage existing codebases and skills. Moreover, Wasm extends beyond the browser, finding applications in server-side computing, edge deployments, and more.
Over 40 programming languages can compile to WebAssembly, including C, C++, Rust, Go, Python, C#, JavaScript, TypeScript, Java, Ruby, PHP, Swift, Kotlin, Scala, and so on.
This flexibility allows developers to choose the most suitable language for their projects and compile it to a performant, portable Wasm module.
Beyond the Browser
While initially designed for web applications, WebAssembly has expanded to various environments:
- Cloud platforms: The Cloud Native Computing Foundation has its own Wasm Working Group, with the goal to make Wasm a first-class alternative to run workloads across Kubernetes and other cloud-native projects. And in the last few years most cloud providers announced support for Wasm modules, such as on AWS and Azure, or using native Kubernetes tools such as KWasm.
- Wasm-native platforms: Platforms like Fermyon enable developers to run Wasm modules on the server to handle HTTP requests, benefiting from fast startup times and efficient resource utilization.
- Edge Computing: Global CDN providers such as Fastly and Cloudflare allow Wasm modules to run on top of edge servers, reducing latency and improving the user experience.
What's next
In the next article, we will dive deeper into the Wasm Component Model.
Unlike standard Wasm modules, Wasm components define types and export functions using WIT (Wasm Interface Type) enhancing portability not only across architectures and operating systems but also across programming languages.
At Edgee, we are working hard to empower developers with Wasm cabilities on the Edgee managed platform. If you're curious to learn more, sign up for the upcoming Edgee Demo Day, reach out on Slack, or check out the Edgee CLI on GitHub.